Table Of Content
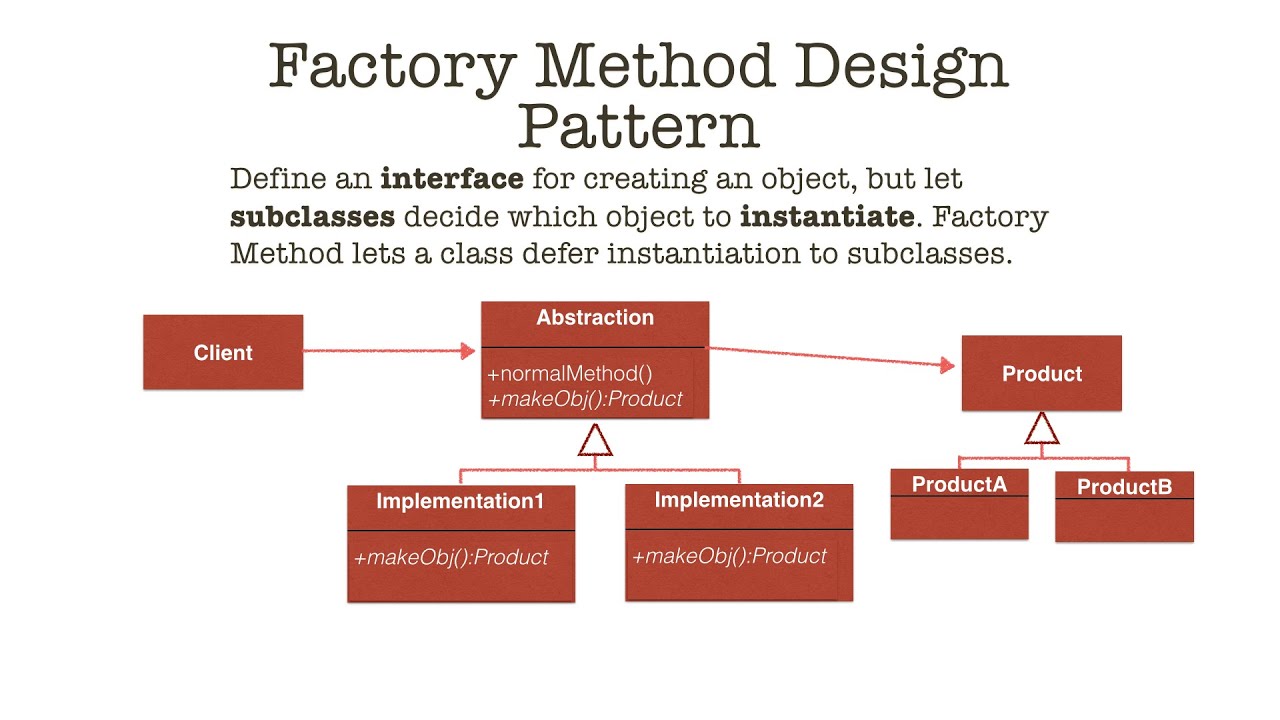
So, the client will get the appropriate object and consume the object without knowing how the object is created and initialized. A good solution is to specialize a general purpose implementation to provide an interface that is concrete to the application context. In this section, you will specialize ObjectFactory in the context of our music services, so the application code communicates the intent better and becomes more readable.
thoughts on “Factory Design Pattern in C#”
They also want to reuse that instance to avoid authorizing the application multiple times. The local service is simpler, but it doesn’t match the initialization interface of the others. Not all situations allow us to use a default .__init__() to create and initialize the objects. It is important that the creator, in this case the Object Factory, returns fully initialized objects. The parameter evaluated in the conditions becomes the parameter to identify the concrete implementation. The first step when you see complex conditional code in an application is to identify the common goal of each of the execution paths (or logical paths).
Factory Design Pattern Sub Classes
For example, in the future, there might be new types of accounts such as Credit, Debit, etc. At that time, we only need to create additional subclasses that extend BankAccount and add them to the factory method. In this step, we’ve created two concrete creator classes, CircleFactory and SquareFactory, which implement the createShape() method to create instances of Circle and Square, respectively. Here, we have two concrete classes, Circle and Square, that inherits from the Shape abstract class.
Add Product Interface
The missing piece is that SerializerFactory has to change to include the support for new formats. This problem is easily solved with the new design because SerializerFactory is a class. Once you make this change, you can verify that the behavior has not changed. Then, you do the same for the XML option by introducing a new method ._serialize_to_xml(), moving the implementation to it, and modifying the elif path to call it. Let’s begin refactoring the code to achieve the desired structure that uses the Factory Method design pattern. Nevertheless, the code above is hard to maintain because it is doing too much.
How to use the flyweight design pattern in C# - InfoWorld
How to use the flyweight design pattern in C#.
Posted: Wed, 08 Jan 2020 08:00:00 GMT [source]
Here, we’ve defined an abstract class Shape with a pure virtual function draw(). This class represents the abstract product that all concrete products must inherit from. I think the explanation in ‘Factory Design Pattern Advantages’ section is quite verbose. So I think the first advantage in the section should be what I said above at least. It's a misconception that design patterns are holy solutions to all problems. Design patterns are techniques to help mitigate some common problems, invented by people who have solved these problems numerous times.
Factory method is a creational design pattern which solves the problem of creating product objects without specifying their concrete classes. Factory Method is a creational design pattern used to create concrete implementations of a common interface. The GoF book describes Factory Method as a creational design pattern.
Step 2: Define Concrete Products (Circle and Square)
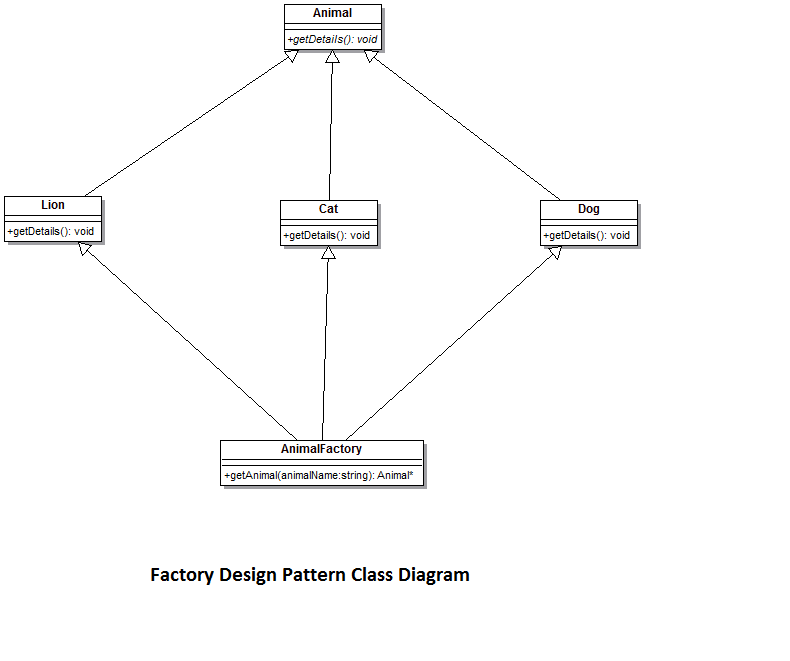
In the method, the Song class uses the serializer object to write its own information without any knowledge of the format. The application can allow the user to select an option that identifies the concrete algorithm. Factory Method can provide the concrete implementation of the algorithm based on this option. You create a song and a serializer, and use the serializer to convert the song to its string representation specifying a format. The final implementation shows the different components of Factory Method. The .serialize() method is the application code that depends on an interface to complete its task.
Real-World Use Cases of the Factory Method Pattern in C++ Design Patterns
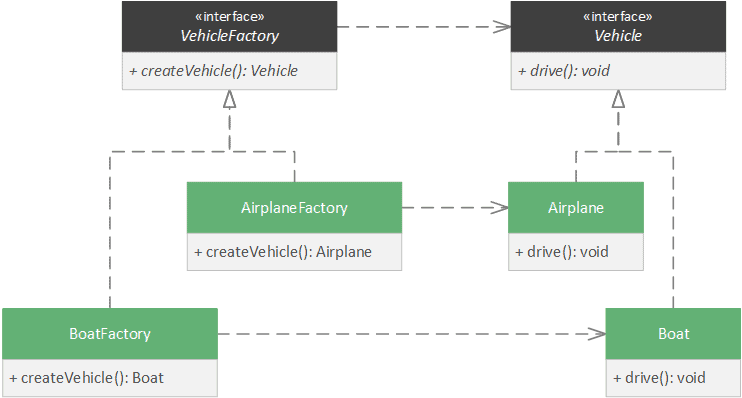
The Factory Design Pattern is a design pattern that provides a single interface for creating objects, with the implementation of the object creation process being handled by a factory class. This factory class is responsible for instantiating objects based on a set of conditions or parameters that are passed to it by the client code. The Factory Design Pattern is a creational design pattern that provides a simple and flexible way to create objects, decoupling the process of object creation from the client code. This pattern can be used to create objects of a single type, or objects of different types based on a set of conditions.
Factory Design Pattern Advantages
Smalltalk-80 MVC in JavaScript - Learning JavaScript Design Patterns [Book] - O'Reilly Media
Smalltalk-80 MVC in JavaScript - Learning JavaScript Design Patterns .
Posted: Mon, 24 Sep 2018 07:18:58 GMT [source]
Make sure to dedicate the necessary time to assessing your technical skills. After you’ve created your account, you will start the quiz right away. Pentalog Connect is your free pass to a large community of top engineers who excel in developing outstanding and impactful digital products. When joining, you receive access to a wealth of resources that will feed your appetite for quality content and your need for professional growth.
Also the examples you mentioned as being present in JDK as example of factory method is also not pure factory pattern. I want you to edit your post so as Correct information reaches the readers. Factory Method is a creational design pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created. It is a creational design pattern that talks about the creation of an object. The factory design pattern says to define an interface ( A java interface or an abstract class) for creating the object and let the subclasses decide which class to instantiate. Factory Method Pattern provides an interface for creating objects but leaves the actual object instantiation to derived classes.
This allows for flexibility in object creation and promotes loose coupling between the creator (client code) and the concrete products. Concrete Creator classes are subclasses of the Creator that implement the factory method to create specific types of objects. Each Concrete Creator is responsible for creating a particular product. In our implementation the drawing framework is the client and the shapes are the products.
A factory (i.e., a class) will create and deliver products (i.e., objects) based on the incoming parameters. The PandoraServiceBuilder implements the same interface, but it uses different parameters and processes to create and initialize the PandoraService. It also keeps the service instance around, so the authorization only happens once. The .register_format(format, creator) method allows registering new formats by specifying a format value used to identify the format and a creator object. The creator object happens to be the class name of the concrete Serializer. This is possible because all the Serializer classes provide a default .__init__() to initialize the instances.
In the example above, you will provide an implementation to serialize to JSON and another for XML. Code that uses if/elif/else usually has a common goal that is implemented in different ways in each logical path. The code above converts a song object to its string representation using a different format in each logical path. You create a song object and a serializer, and you convert the song to its string representation by using the .serialize() method. The method takes the song object as a parameter, as well as a string value representing the format you want.
This implementation represents an alternative for the class registration implementation. This implementation is the most simple and intuitive (Let's call it noob implementation). The problem here is that once we add a new concrete product call we should modify the Factory class. Of course we can subclass the factory class, but let's not forget that the factory class is usually used as a singleton.
All the shapes are derived from an abstract shape class (or interface). The Shape class defines the draw and move operations which must be implemented by the concrete shapes. Let's assume a command is selected from the menu to create a new Circle.
By abstracting the creation process into separate factory methods, you can write more maintainable, modular and testable code. It is a fundamental patten to have in your arsenal when designing object-oriented systems, offering a solution to the often complex problem of object instantiation. If the factory is an interface - the subclasses must define their own factory methods for creating objects because there is no default implementation.
No comments:
Post a Comment